Puzzle: US City With Only 3 Letters
The Sunday Puzzle Challenge:
The name of what well-known U.S. city, in 10 letters, contains only three different letters of the alphabet? Puzzle URL
Synopsis
Fun and Simple! You can get the census place name file from the US Census Bureau.
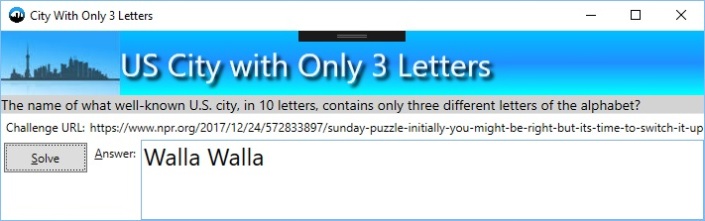
Techniques Used
Code to Solve the Puzzle
private void btnSolve_Click(object sender, RoutedEventArgs e) {
string cityFile =
@"C:\Users\User\Documents\Puzzles\Data\AmericanPlaces2k.txt";
using(StreamReader sr = File.OpenText(cityFile)) {
while(sr.Peek() != -1) {
string aLine = sr.ReadLine();
//File is fixed-width, city starts in column 9
string cityName = aLine.Substring(9, 30)
.TrimEnd();
//Place names end with town/city/CDP; remove it now:
if (cityName.EndsWith(" town"))
cityName = cityName.Substring(0, cityName.Length - 5);
else if (cityName.EndsWith(" CDP"))
cityName = cityName.Substring(0, cityName.Length - 4);
else if (cityName.EndsWith(" city"))
cityName = cityName.Substring(0, cityName.Length - 5);
//Remove spaces and other non-letters:
var letters = cityName.ToLower()
.Where(c => char.IsLetter(c));
//Check if the city has 10 letters and a distinct count of 3
if (letters.Count() == 10) {
int letterCount = letters.Distinct().Count();
if (letterCount == 3) {
txtSolution.Text += $"{cityName}\n";
}
}
}
}
}
Any Tricky Parts?
When you use Linq on a string variable, it treats your variable as an array of char. That is how I can, for example, execute this line of code:
var letters = cityName.ToLower().Where(c => char.IsLetter(c));
In the code above, ‘c‘, refers to a char in the city name. Note that the result above, ‘letters‘, has data type ‘IEnumerble<char>’. I.e., everything is a char when you use Linq on a string.
Download the Code
You can download the code from my DropBox account. To do so, you will need to create a free account. If you run the downloaded code, you will need to adjust the path to the city name file. I included that file in the download.