Month: March 2015
WPF – Animate Textboxes to Highlight Bad Data
WPF makes extremely good UIs (i.e. easy to use and attactive), but most programmers don’t take advantage of the power at their fingertips. One common scenario is providing immediate feedback when your users mess-up. I like to know immediately, not when I try to click the button to submit. Because I hate it when I don’t realize I made a mistake until long after the fact!
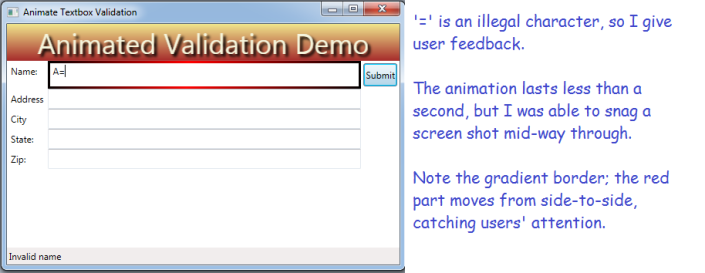
The Technique
- Set-up validation for multiple textboxes with the same code
- Using delegates to reduce your code!
- Build a border animation
- Using a gradient
- Your gradient will use a moving middle stop, generating something like a wiping effect
- Every time the textbox change event fires, call your validation on users’ text;
- If bad, display your fancy animation, and set the tool tip and status bar message
The result looks (IMHO) pretty slick and is hard. I prefer it to the standard technique (IDataErrorInfo) for scenarios when I have several textboxes with the same validation rules, because it is less work overall.
The Fun Part – Animate Your Border!
public static void ReddenTextboxBorder(TextBox txt, MainWindow theMainWindow) {
//Starting by defining 3 gradient stops; the middle one will move from left to right
GradientStop stop1 = new GradientStop(Colors.Black, 0);
GradientStop stop2 = new GradientStop(Colors.Red, 0);
//Since we created the stop 'on-the-fly', we need to register the name to animate
theMainWindow.RegisterName("stop2", stop2);
GradientStop stop3 = new GradientStop(Colors.Black, 1);
//We will use the brush for the border being animated, tell it all 3 stops:
LinearGradientBrush gradBrush = new LinearGradientBrush(new GradientStopCollection { stop1, stop2, stop3 });
txt.BorderBrush = gradBrush;
//The animation will go from 1% to 99% over .3 second time period:
DoubleAnimation ani = new DoubleAnimation(.1, .99, new Duration(TimeSpan.FromMilliseconds(300)));
//When we hit the right border, bounce back to the left again:
ani.AutoReverse = true;
//Storyboard helps connect the animation parts
Storyboard sb = new Storyboard();
Storyboard.SetTargetName(ani, "stop2");
Storyboard.SetTargetProperty(ani, new PropertyPath(GradientStop.OffsetProperty));
sb.Children.Add(ani);
//After the animation is don, set the border to pure red (no gradient)
sb.Completed += delegate(object sndr, EventArgs evtArg) { txt.BorderBrush = new SolidColorBrush(Colors.Red); };
sb.Begin(theMainWindow);
//By unregistering the name, I release memory and become confident I can re-run without issues
theMainWindow.UnregisterName("stop2");
}
At this point, we have a method to animate any textbox, so long as it inhabits our main form. It looks cool and tells the user they messed-up with minimal pain. Now, let’s hook-up several textboxes to use our new method:
Connect the Animation to Your Validation
private void SetUpRequiredFieldValidation()
{
//An array of textboxes which must be populated before user
//can click the button to do some work
TextBox[] _RequiredTextBoxes = new[] { txtName,
txtAddress,
txtCity,
txtState,
txtZip
};
//Hook-up a TextChanged event for every textbox in the array;
foreach (TextBox tb in _RequiredTextBoxes)
{
//Dynamically declare an event to fire for each textbox using a delegate
tb.TextChanged += delegate(object sender, TextChangedEventArgs e) {
//'ValidIdentifier' (omitted for clarity) is my Regular Expression that checks
//whether the textbox text is valid. You could substitue
/your own validation logic here, such as 'String.IsNullOrWhitespace(tb.Text)'
if (Utility.ValidIdentifier.IsMatch(tb.Text))
{
//Omitted for clarity - clear the color/tooltip/border thickness etc.
ResetTextboxBorder(tb);
}
else
{
tb.BorderThickness = new Thickness(3);
ReddenTextboxBorder(tb, this);
tb.ToolTip = "Invalid name";
//Display some information in the status bar:
stbMessage.Text = "Learn to type, user!";
}
};
}
}
Here, I build an array containing all the textboxes I wish to validate. I then loop through them, and for each textbox, hook-up a TextChanged event. That event
- Checks if the uers’ text is valid, if so
- Clear any previous effects from the textbox
- Otherwise, animate the border and set the tool tip
Summary
Animation makes your applications easier to use and less frustrating. More importantly, apps with animated feedback require less training. If you have a lot of users, training costs can be significant, so you are really cutting-down on the cost to run your app. Your user manager should love you when you save them costs! Maybe you will be recognized for writing superior code! BTW, you could do this in HTML/ASP or other web technologies, but not as slickly nor as easily! WPF makes vastly superior UIs!